Fix Github Actions Error on XCode 14
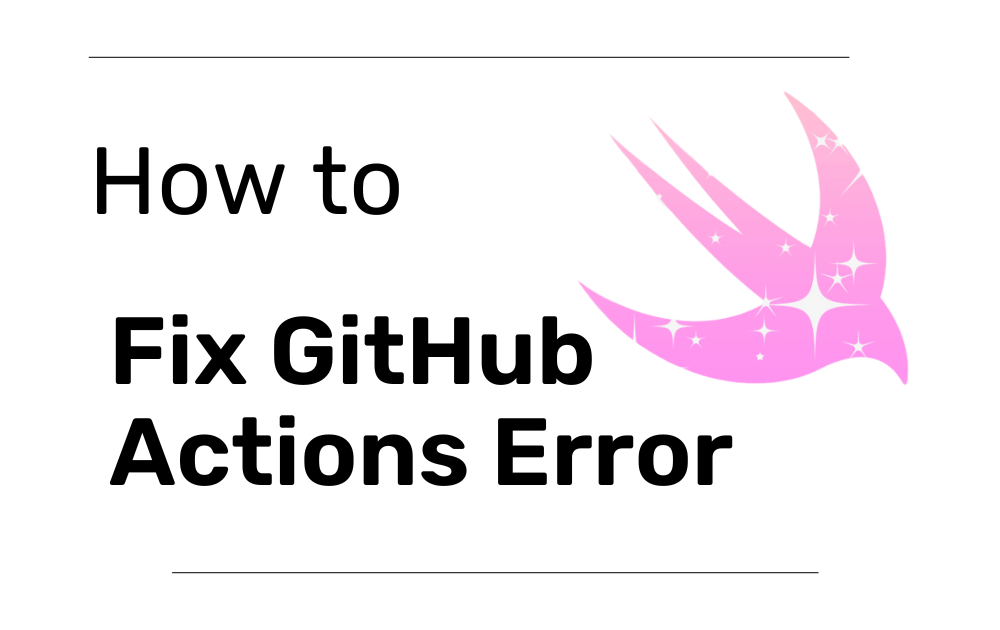
If you're using Github Actions with XCode 14, you probably need to make some changes to your project to avoid getting errors on your pipeline. Especially if the Xcode version you're using is different than the one that the pipeline uses (in my case I was using XCode 15).
In this post, I'm going to explain how to fix this error that seemingly makes no sense.

From iOS 17 and forward some features were added and in particular, making this method public from UIViewController, the viewIsAppearing.
This means that when GitHub actions are running, it's using XCode 14 against your code.
On iOS 16.1 it wasn't possible to override the viewIsAppearing so that's why it's giving an error on the pipeline and when you're XCode 15+ the tests are passing. Most of this code was using Objective-C back then.
Solution
If the project you have does not have a Bridging Header you may need to create a header file and set this Bridging Header under Objective-C Bridging Header on your target.
On this header file, you define an extension or category with the viewIsAppearing method signature.
#import "Availability.h"
#if defined(__IPHONE_17_0)
#warning "UIViewController+UpcomingLifecycleMethods.h is redundant when compiling with the iOS 17 SDK"
#else
@import UIKit;
@interface UIViewController (UpcomingLifecycleMethods)
/// Called when the view is becoming visible at the beginning of the appearance transition,
/// after it has been added to the hierarchy and been laid out by its superview. This method
/// is very similar to -viewWillAppear: and is always called shortly afterwards (so changes
/// made in either callback will be visible to the user at the same time), but unlike
/// -viewWillAppear:, at the time when -viewIsAppearing: is called all of the following are
/// valid for the view controller and its own view:
/// - View controller and view's trait collection
/// - View's superview chain and window
/// - View's geometry (e.g. frame/bounds, safe area insets, layout margins)
/// Choose this method instead of -viewWillAppear: by default, as it is a direct replacement
/// that provides equivalent or superior behavior in nearly all cases.
///
/// - SeeAlso: https://developer.apple.com/documentation/uikit/uiviewcontroller/4195485-viewisappearing
- (void)viewIsAppearing:(BOOL)animated API_AVAILABLE(ios(13.0), tvos(13.0)) API_UNAVAILABLE(watchos);
@end
#endif
This code was implemented by Liam Nichols on this blog post: https://liamnichols.eu/2023/06/12/view-is-appearing.html
Now you can add this file to the Objective-C Bridging Header on your target as follows.
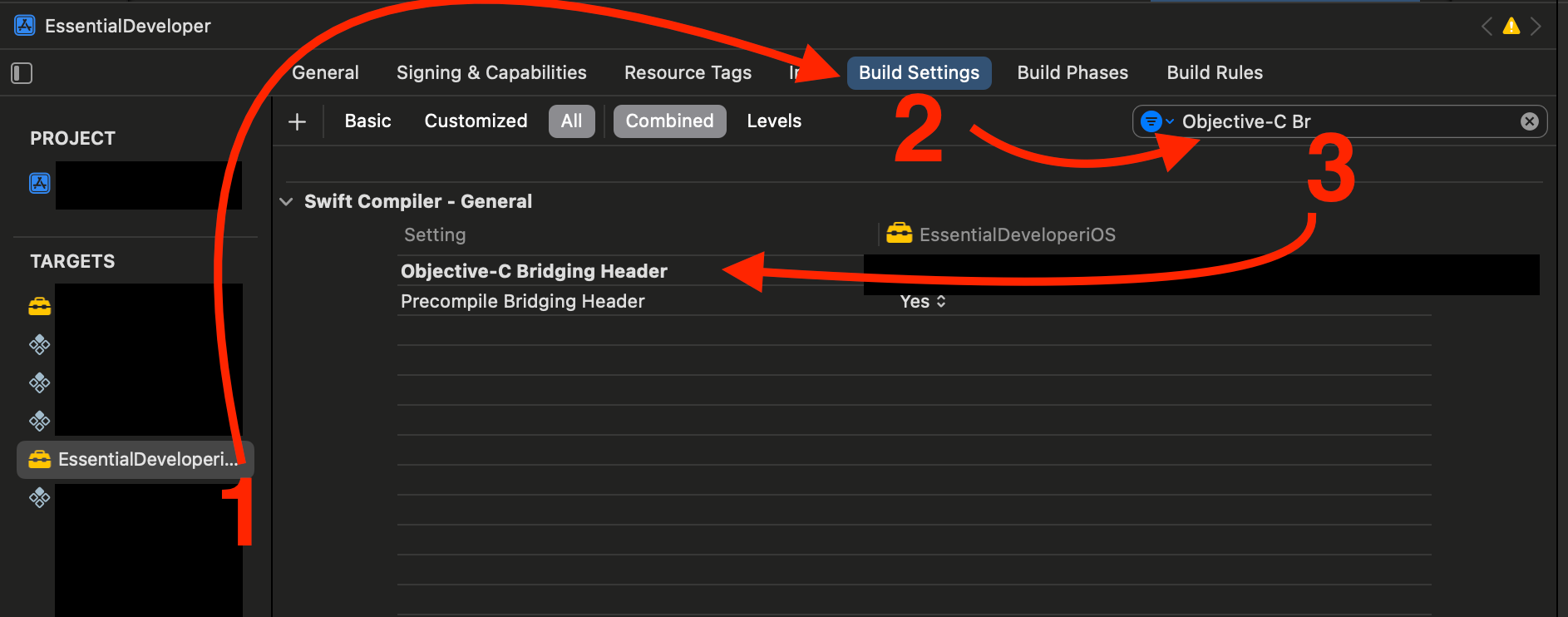
Click on the option and insert the following path to your header file.
$(SRCROOT)/Path/To/headerFile.h
After this, you can use the override method signature on your code and still have the GitHubActions workflow pass!
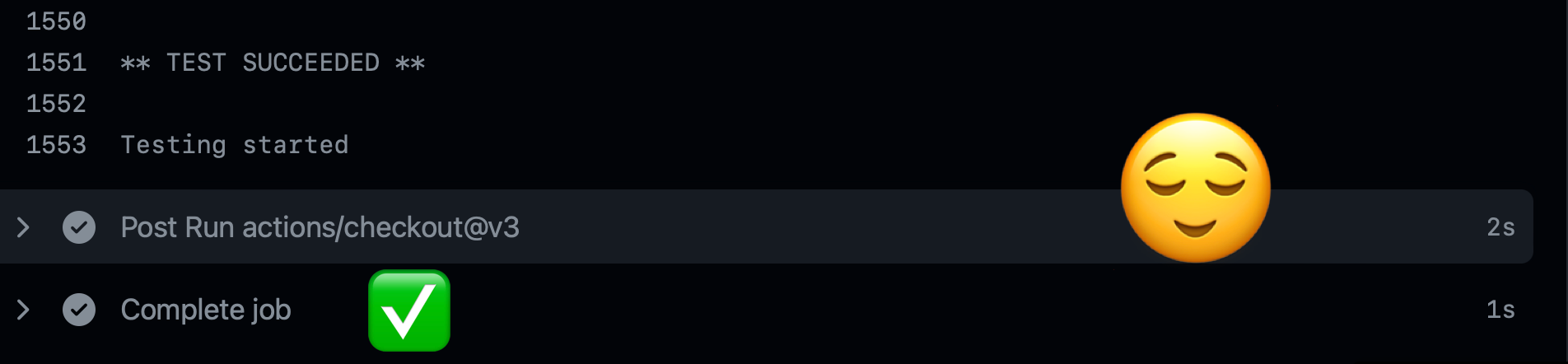
Big thank you to Liam Nechols, they created a post back on Jun 2023 with the Bridging Header solution that was used in this post.
Sharing this post will help other people with the same issues and you're also motivating me to create more content. Thank you for reading.
References
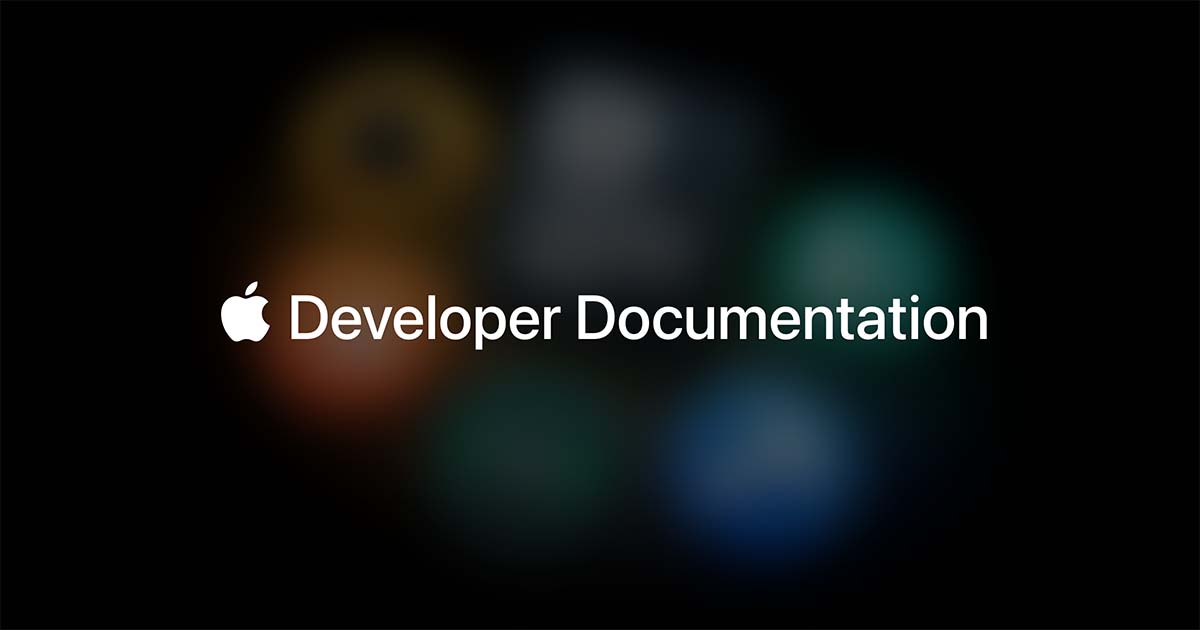
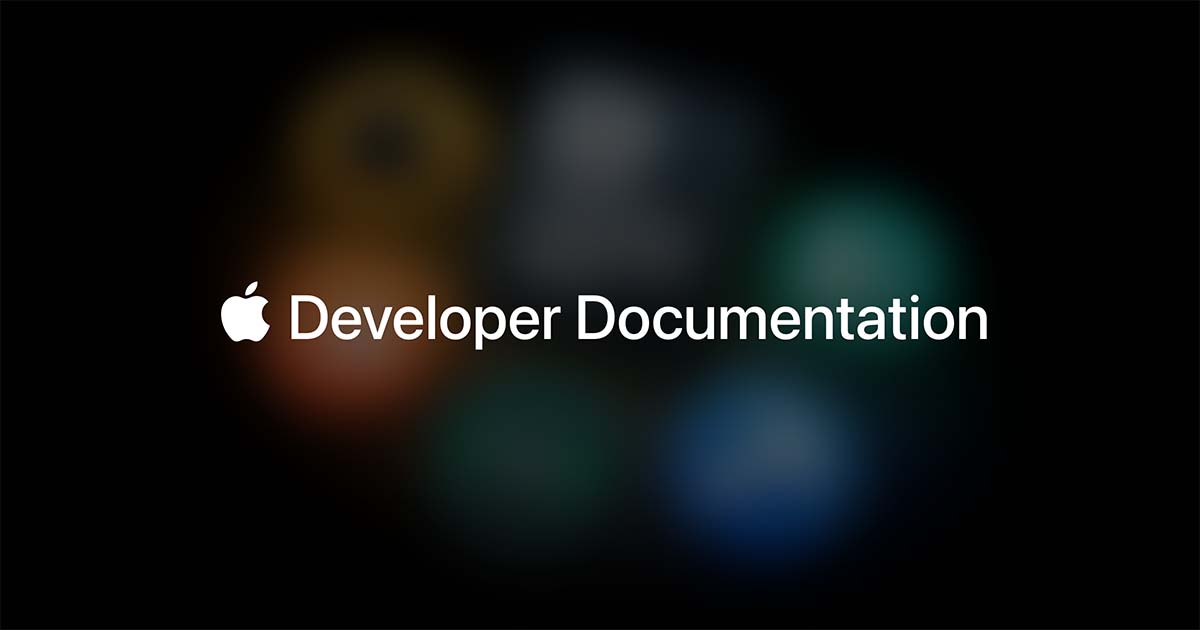
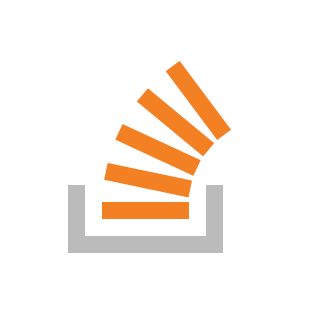
Thank you for taking the time to read this article.
If you enjoyed my work and want to stay updated on future projects, don't hesitate to connect with me on GitHub or LinkedIn. Thank you 🙏🏻