Setup GitHub Actions on Your iOS Project
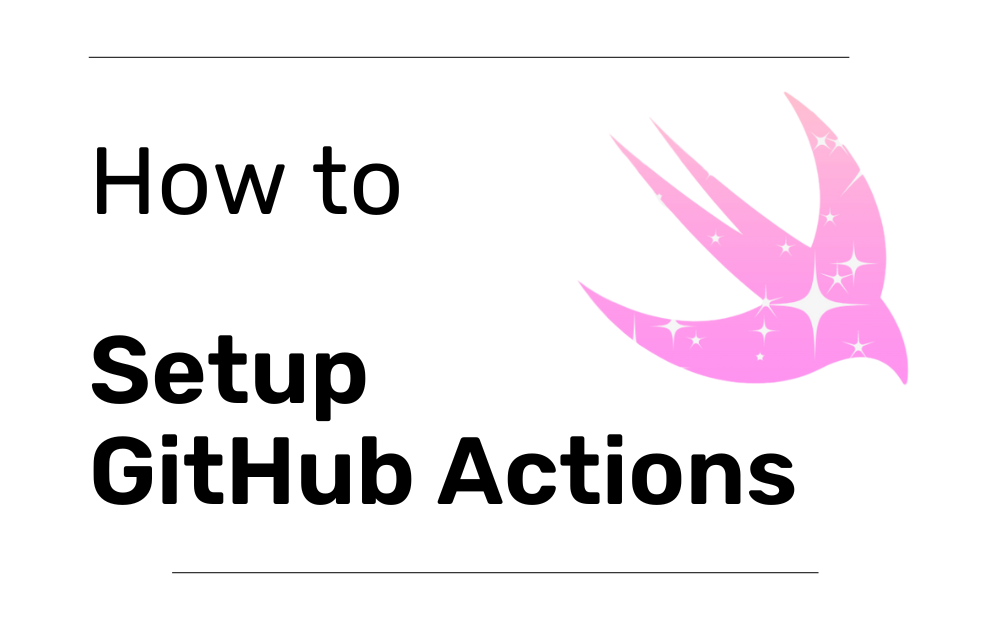
Introduction
With the introduction of Github Actions it's now easier than ever to implement CI on your project. You can even use the latest version of iOS on the free tier!
In this post we're going to go briefly through the setup and you can visit my GitHub to check my configuration.
Before you Read
Make sure you have a scheme that tests and builds what you want. In my case I had a separate scheme just for the CI.
Setup
Click on the Actions tab under your GitHub project.

- Search for Swift if you want to have a basic setup of the yml file. However this is optional, you can create one from scratch as well.
- Commit the file setup to your project.
Setting Up the File
Now that you have the yml file on your project you need to set the following:
- Name of the job (in my case was CI)
- When will it run, this is based on your needs. It can run when there are pushes to the main branch or when a pull request opens to the main branch
So, for now we have:
name: CI
on:
push:
branches: [ "main" ]
pull_request:
branches: [ "main" ]
Creating a Job
For the last step it's necessary to create the job that it will be run.
This job should have an appropriate name and it should state the macOS version as one of the first arguments.
Before you insert the step to build and test your project you need to select your Xcode version. You can do this by using
xcode-select -switch /Applications/Xcode_VERSION.NUMBER.app
Finally, you just need to setup the job. In my case I just wanted to build and test a MacOS application so this step is named Build And Test MacOS with the following command:
xcodebuild clean build test -project PATH-TO-YOUR/App.xcodeproj -scheme "NAME_OF_SCHEME_ON_YOUR_PROJECT" CODE_SIGN_IDENTITY="" CODE_SIGNING_REQUIRED=NO
name: CI
on:
push:
branches: [ "main" ]
pull_request:
branches: [ "main" ]
jobs:
build-and-test:
runs-on: macos-13
steps:
- uses: actions/checkout@v4
- name: Select Xcode
run: sudo xcode-select -switch /Applications/Xcode_15.2.app
- name: Xcode version
run: /usr/bin/xcodebuild -version
- name: Build and Test MacOS
run: xcodebuild clean build test -project Project/MyProject.xcodeproj -scheme "CI_macOS" CODE_SIGN_IDENTITY="" CODE_SIGNING_REQUIRED=NO
Bonus: Creating a Job for iOS Schemes
If the scheme you want to run is not platform agnostic you need to specify the simulator version of the iPhone.
So you create another step on the job you already have and specify the iPhone simulator using this command:
-sdk iphonesimulator -destination "platform=iOS Simulator,name=Name_Of_iPhone_On_Xcode,OS=XX.XX.XX"
So in the end you'll have something like this:
name: CI
on:
push:
branches: [ "main" ]
pull_request:
branches: [ "main" ]
jobs:
build-and-test:
runs-on: macos-13
steps:
- uses: actions/checkout@v4
- name: Select Xcode
run: sudo xcode-select -switch /Applications/Xcode_15.2.app
- name: Xcode version
run: /usr/bin/xcodebuild -version
- name: Build and Test MacOS
run: xcodebuild clean build test -project Project/MyProject.xcodeproj -scheme "CI_macOS" CODE_SIGN_IDENTITY="" CODE_SIGNING_REQUIRED=NO
- name: Build and Test iOS
run: xcodebuild clean build test -project Project/MyProject.xcodeproj -scheme "CI_iOS" CODE_SIGN_IDENTITY="" CODE_SIGNING_REQUIRED=NO -sdk iphonesimulator -destination "platform=iOS Simulator,name=iPhone 15 Pro Max,OS=17.0.1" ONLY_ACTIVE_ARCH=YES SWIFT_TREAT_WARNINGS_AS_ERRORS=YES OTHER_SWIFT_FLAGS="-D ED_SKIP_SWIFT_FORMAT"
You can see an example of a yml file similar to the one shown here on my GitHub.
Thank you to Essential Developer for the video on how to setup a CI with GitHub Actions.
Thank you for reading!
References
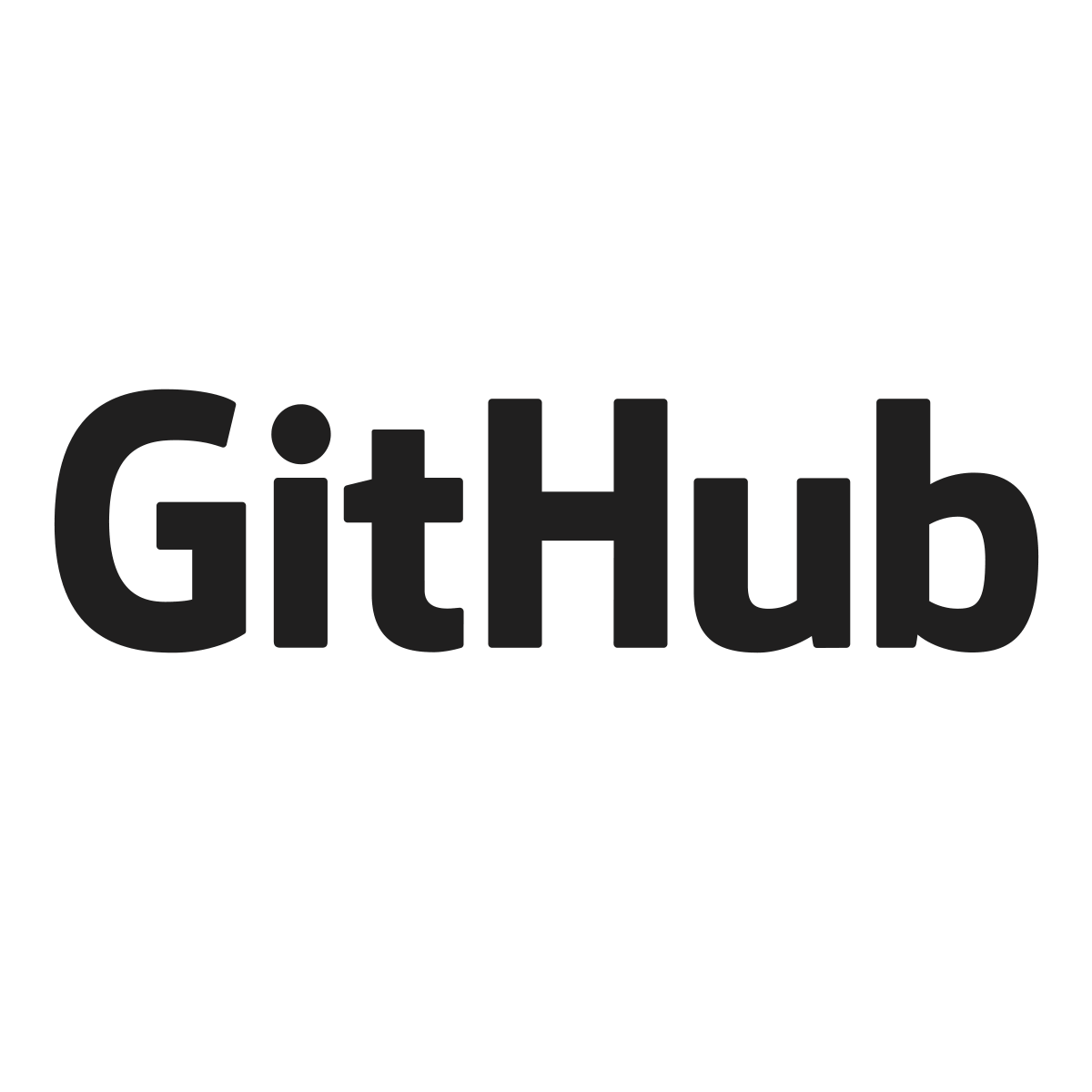

Thank you for taking the time to read this article.
If you enjoyed my work and want to stay updated on future projects, don't hesitate to connect with me on GitHub or LinkedIn. Thank you 🙏🏻