Swift 5.9: Copyable Syntax
Introduction
With the introduction of Swift 5.9 a lot of new and exciting features were introduces such as Macros, improvements on Swift-Syntax and many more.
In this article I want to go over a term that I hadn't heard before called noncopyable structs and enums, which is having a lot of buzz around lately.
And since I'm sure I'm not the only one that is wondering everytime I'm in a forums: "What is a Copyable?" I decided to share my findings with you.
Ownership
First things first, let's talk about the big picture: ownership. According to swift official documentation, ownership is about helping developers improve memory management in code that relies on performance [1].
Right out of the bat this makes sense, most of the memory handling is being done by the compiler but if we can improve the performance on certains situations why shouldn't we?
And due to this Swift introduced noncopyable for structs and enums.
I recommend you check out the full proposal page to learn more about this.
Copyable
So what is a copyable?
Up until now, all existing types in swift are copyable which means that you can create multiple instances of any value of the type [3].
The problem arises with structs and enums which are value types [4]. One of the biggest disadvantages of value types is they do not hold the same reference in memory when copied.
This means that if you copy one object that is a value type, the second object that you get is exactly the same as the first one but they are not referencing the same element in memory.
Here's what I mean:
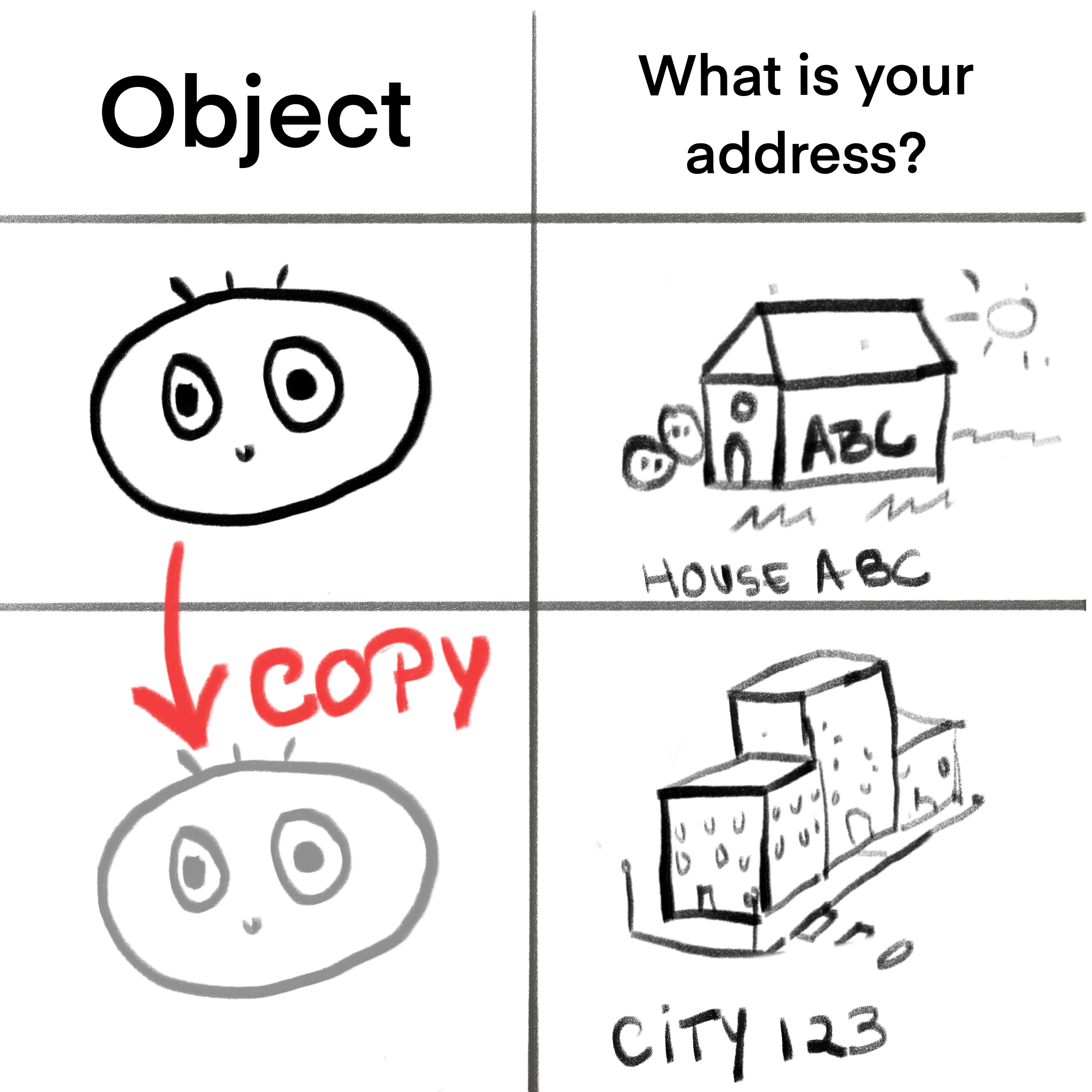
But when you're using reference types you won't have this problem, the original and the copy will have the same reference in memory.
On the other hand, if you use reference types you need to take into account reference counting. This is a strategy to make sure objects that are reference types are allocated in memory correctly.
So on one hand you have value types and on the other reference types, where does noncopyable come in?
Noncopyable
With Swift 5.9 it was introduced a an hybrid for structs and classes: the non copyable.
This means that if you explicitily state that the value type you're using is non-copyable, this means that it will have a unique ownership (similar to what happens with classes).
Why are we using class behaviour on structs and enums, isn't it better to use classes instead?
The big advantage here is that you don't need to worry about allocation with noncopyable types.
The value type is still allocated in the stack frame throught it's file descriptor ID and or aggregates the value that uniquely owns the instance [3].
How to Use
To make a value type noncopyable
you need to explicitily remove the Copyable
ability by adding the syntax ~Copyable
.
Let's see an example.
struct Fish {
let tail: TailType
let habitat: String
}
enum TailType {
case long
case short
case nonexistent
}
As I said before all structs and enums are Copyable
by default.
So how can you make it noncopyable
?
struct Fish: ~Copyable {
let tail: TailType
let habitat: String
}
Conclusion
Swift 5.9 introduced more tools so that you can have more control over memory management. Every year there is a bigger and bigger push from Apple to use value types so the introduction of noncopyable
syntax helps close the gap since it adds one of the biggest advantages of using reference types: when copied the two instances point the same reference to memory.
Hopefully, this article has shed some light on the concept and its impact. For a deeper dive, I highly recommend checking out the official Swift proposal.
Thank you for reading.
Answering Your Questions
Today's question comes from Eric and they said:
Hey Vera, do you feel like there are fewer opportunities for mobile devs right now? It seems like everyone is on the hunt for a job.
Good question—it’s tough seeing so many people in the industry job hunting right now.
I might have an unpopular take, but I don’t think mobile dev jobs are actually decreasing. Mobile development will always be in demand as people rely on their phones more than ever.
That said, the industry goes through phases—some periods have more opportunities than others (think 2020 vs. now).
One thing that really helped me land a job was having a mentor. If you have the financial means, investing in mentorship can be a game-changer for your career.
Ana Nogal was my mentor and I can't recommend her enough. She has few spots available to mentor other engineers, so if you want to take the next step in your career consider a mentorship with her.
And if you use my code VERADIAS1020 you can get 10% off 🤫
Thank you for your question!
Have a chance to be featured in the next post by sending me an e-mail. 👇
References
[1] Swift 5.9 release notes: https://www.swift.org/blog/swift-5.9-released/
[2] Swift Macros Deep Dive: https://codingwithvera.com/deep-dive-into-macros/
[3] Noncopyable structs and enums: https://github.com/swiftlang/swift-evolution/blob/main/proposals/0390-noncopyable-structs-and-enums.md
[4] Value Types and Reference Types: https://codingwithvera.com/struct-vs-class-which-one-do-i-need/