What the **** is an App Lifecycle?!
Every app has a life cycle from when the user taps to open it to the end when the user chooses to close it. In this article, I'll review the different states of an app's life cycle and the methods for utilizing them.
Every app has a lifecycle from when the user taps to open it to when the user chooses to close it.
But how and why should we, as developers, take advantage of these different states?
In this article, I'll review the different states of an app's lifecycle and the methods for utilizing them.
Definition
The concept of lifecycle is much broader than software development. From Merriam-Webster, the definition is as follows [1]:
The series of stages in form and functional activity through which an organism passes between successive recurrences of a specified primary stage.
Or, in Leyman's terms, all of the stages between the beginning and the end of something that, in this case, is an app.
However, this is not even a new concept in mobile development. Take, for example, our other mobile sibling, Android, with vast documentation on the topic [2]:
Within the lifecycle callback methods, you can declare how your activity behaves when the user leaves and re-enters the activity. (...)
(..) For example, if you're building a streaming video player, you might pause the video and terminate the network connection when the user switches to another app. (...)
Now, we can understand the different stages of an app's lifecycle.
Lifecycle Stages
Before we discuss the different stages, I would like to give you the definitions of foreground and background.
Foreground
Something is visible to you. An app is on your device's screen.
Background
It's not in front of you; the app may be running, but it's not actively in use or visible on your device.
Now that we have these two definitions, we can learn more about the different stages.
Stages
The app's first stage starts with the Not Running [3] state.
Not Running
As the name says, the app is not running in either foreground or background.
How can you reach this state:
- You terminated an app [3];
- An app has not been launched yet [3].
If you click to open an app, it doesn't immediately go to active; it first goes through the Inactive [3] state.
Inactive
The app is running in the foreground but is not responsive to the user (you can tap on it, but nothing happens).
How can you reach this state:
- The app is transitioning from one state to another (opening the app goes from not running to active) [3].
After going through the inactive state, the app may transition to a stage that is accepting user input, such as the Active [3] state.
Active
The app is running in the foreground and receiving events. It's responsive and has no special restrictions [3].
How can you reach this state:
- Open an app and wait for the launch to finish. You are active when the app becomes responsive and in the foreground.
When you finish interacting with the app, you may open another. In that case, the first app will go to the background state.
Background
The app is running and maybe executing code, but it's not visible on the screen [3].
How can you reach this state:
- Open one app, and after finishing it, go to your home. The app is in the background.
Finally, if the app has finished loading all tasks, the system will suspend it [4].
Suspended
The app has finished running all of the tasks. The app is still in memory but isn't running code [4].
But why would the system want to keep apps in memory?
Resume them as quickly as possible when you open them again [4].
How can you reach this state:
- Open one app, let it go to the active state, and then open another. The first app will be suspended.
Now that we have cleared all states let's examine a few examples to understand how it works better.
Examples
Now imagine you're opening an app, what is the state of the app before you tap on it? The state is Not Running.
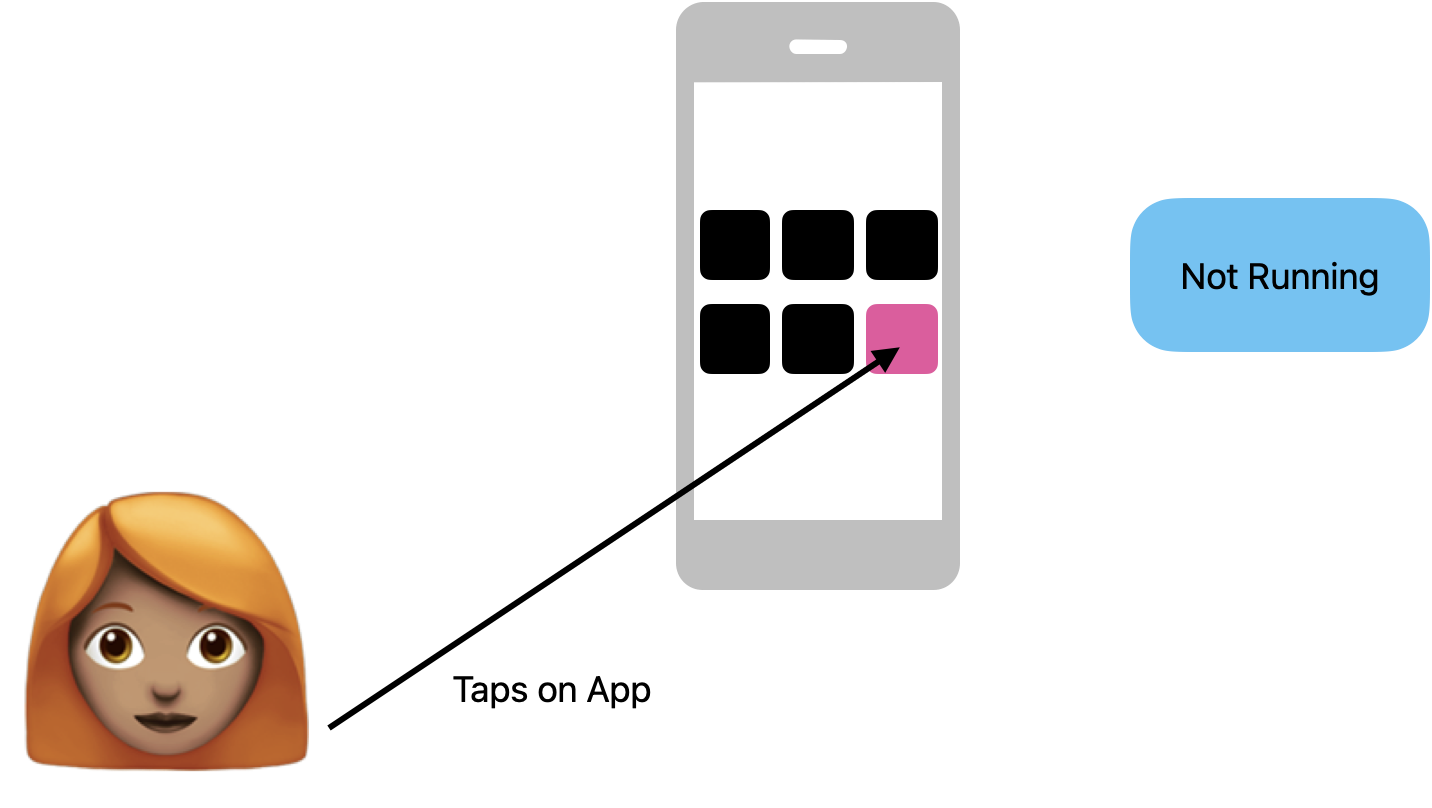
What is the state when you open an app? If you tap on it and it's not responsive, it's Inactive.
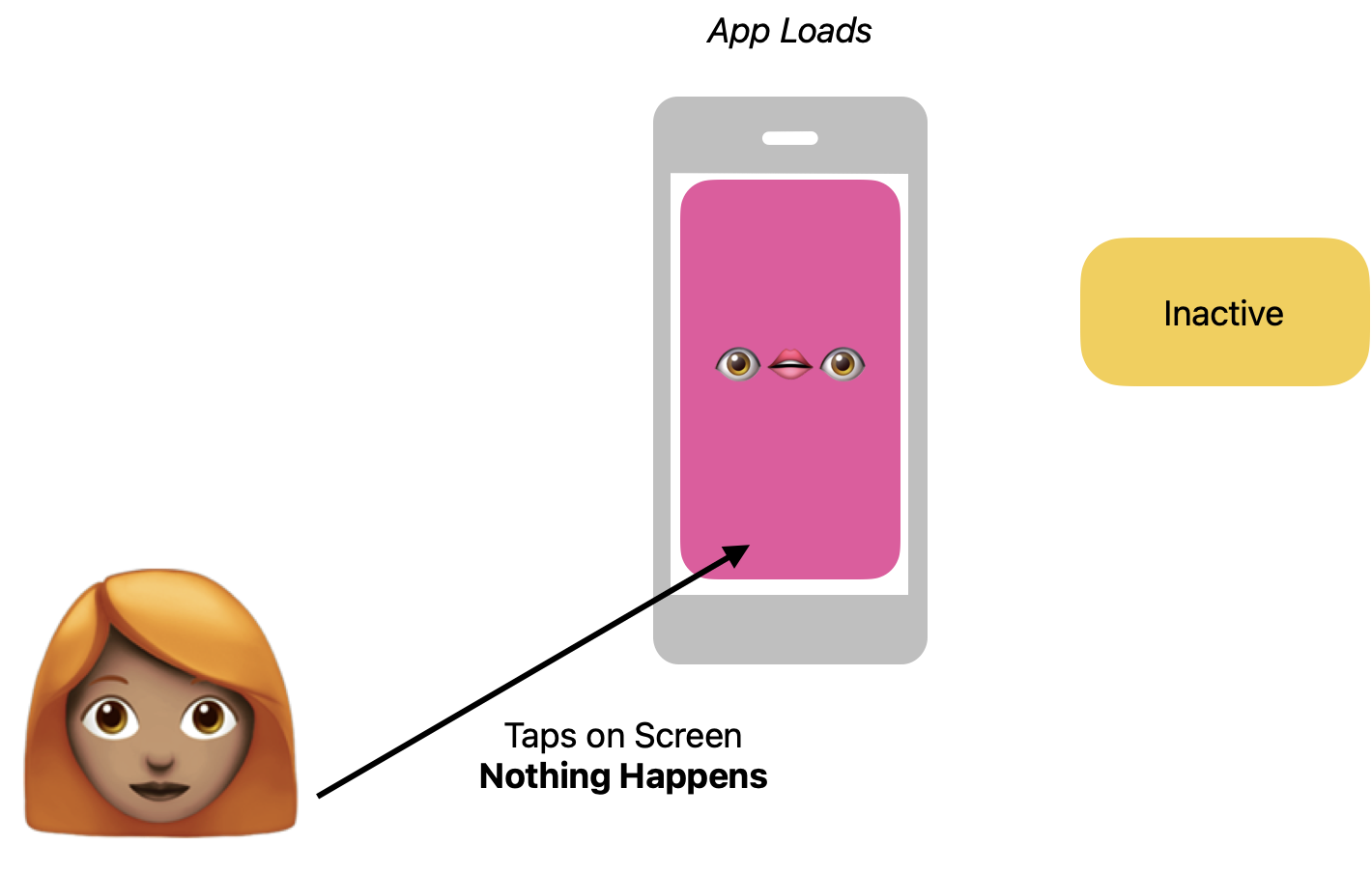
Shortly after the app loads, you can interact with it, so that is an Active state.
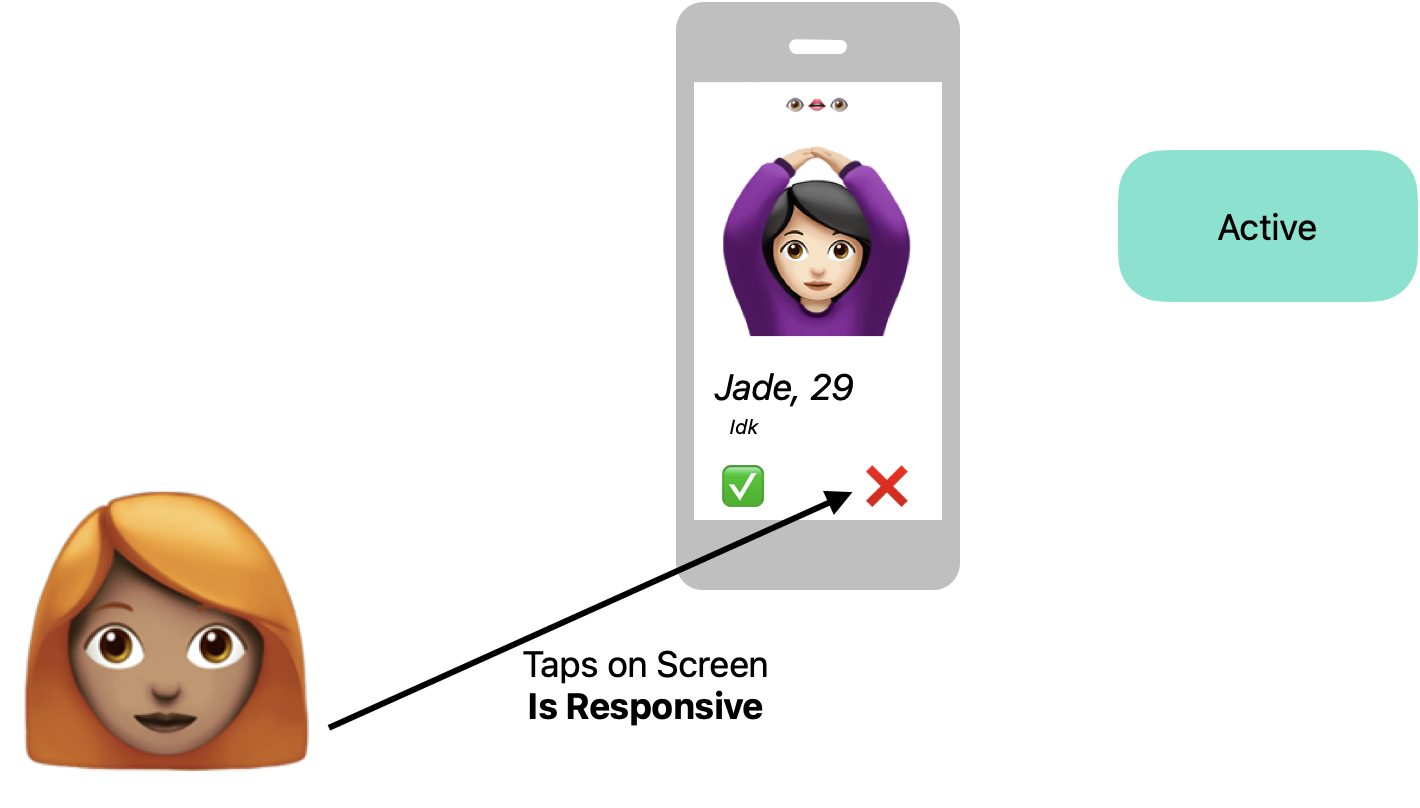
What if you receive a notification when you're interacting with the app? What is the state then? In that case, the app will be Inactive.
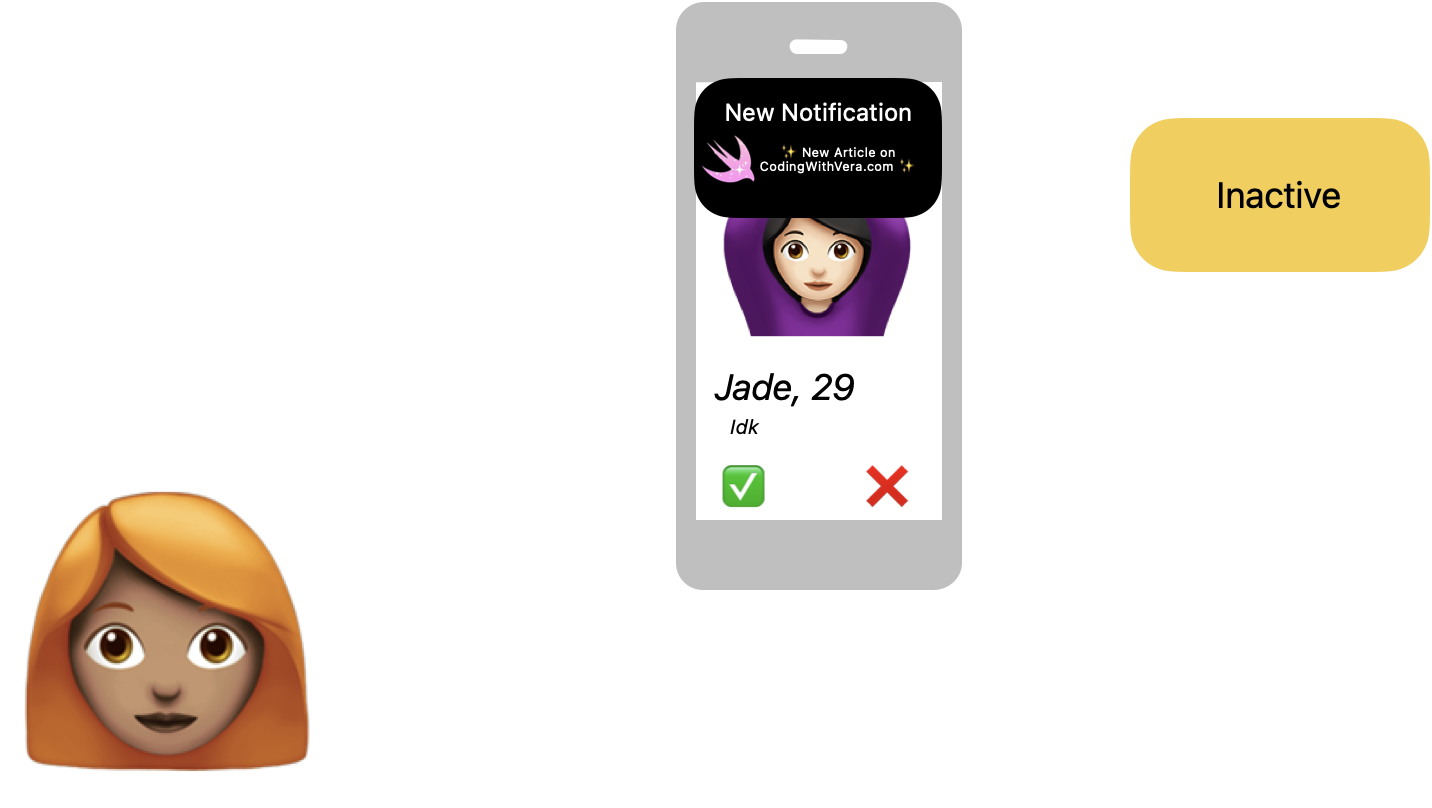
Finally, when you're done, you close the app, and it briefly goes through the Inactive and Background state and then back to the Not Running.
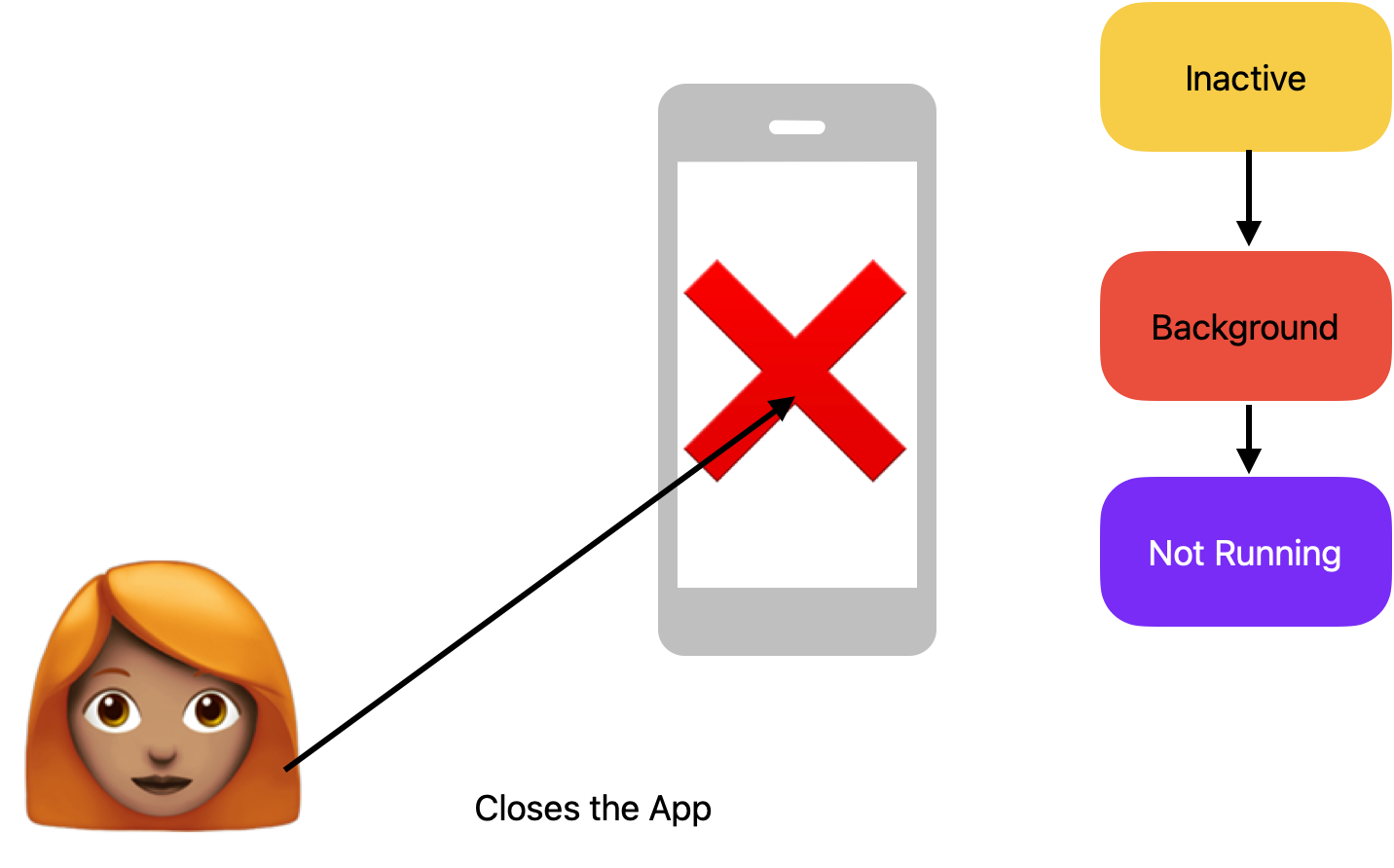
Now that we have consolidated the different states, we can move on to how UIKit handles them through the delegate.
Handling the Lifecycle
You should use the UlApplicationDelegate protocol to handle the app's various states throughout its lifecycle [5].
Did Finish Launching
The first method that is called when the app is finished launching is application(_:didFinishLaunchingWithOptions:)
[6].
When does it happen:
- Right after the launch of the app
Will Resign Active
This method applicationWillResignActive(_:)
[8] is called when you move from an active state to an inactive state or leave the background [7].
When does it happen [7]:
- You're quitting the app;
- You received a notification (and it's presented over the app).
Did Become Active
The app went from inactive to an active state. The method that is called is applicationDidBecomeActive(_:)
[9].
When does it happen [10]:
- You just launched an app;
- You ignored a notification (you swiped up on the notification and went back to the app).
Did Enter Background
It is called right after the applicationDidEnterBackground(_:)
[11] but the app is already in the background [12].
When does it happen [12]:
- You quit the app. You only spend a few seconds in this state before removing the app from memory.
Will Enter Foreground
The method applicationWillEnterForeground(_:)
[13] from a transition from background to an active state before the applicationDidBecomeActive(_:)
is called [12].
Will Terminate
The function applicationWillTerminate(_:)
[14] is called when the app is about to be cleared from memory. Very similar to applicationDidEnterBackground(_:)
but there are differences.
Will Terminate vs. Did Enter Background
Depending on the type of app you should use applicationWillTerminate(_:)
or applicationDidEnterBackground(_:)
.
Ask yourself this question:
Is your app allowed to execute code in the background? Like playing music or handling video calls? [10]
Then you should use applicationDidEnterBackground(_:)
.
Else, you should use applicationWillTerminate(_:)
.
Conclusion
Now that you know the different states and how the UlApplicationDelegate delivers functions that you can use to your advantage, we can apply the different functions using the previous illustrations.
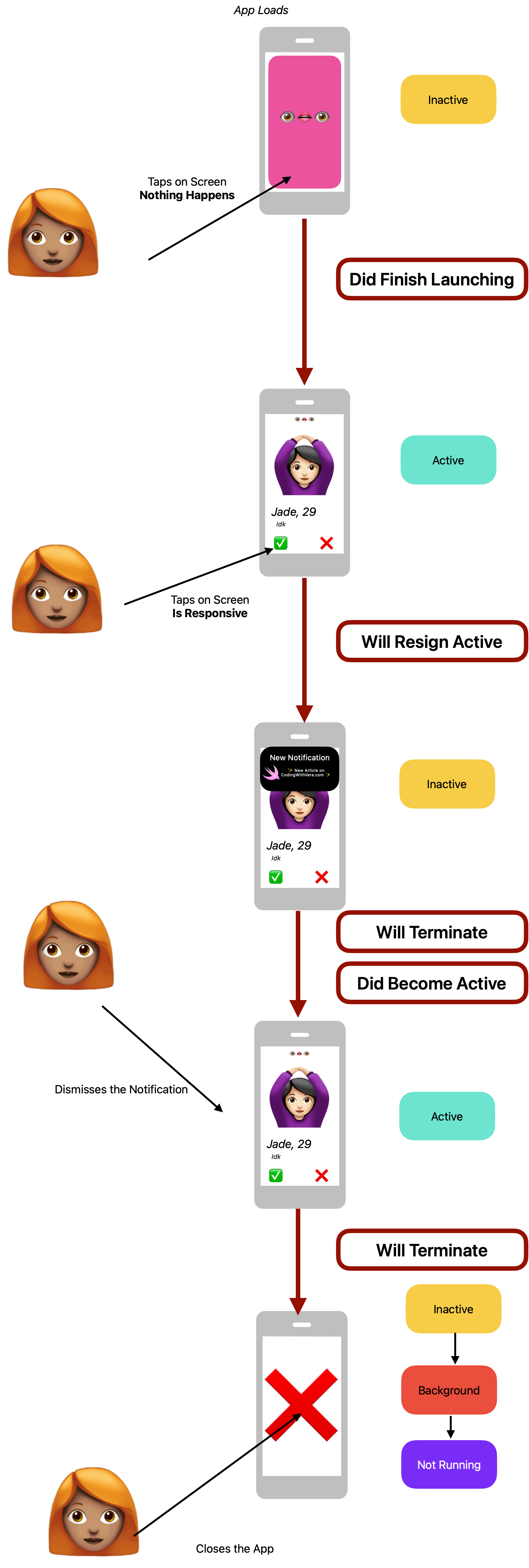
Now, you know more about the app's lifecycle and how to use it for different operations.
I hope you enjoyed this article.
It brings me great joy to create articles and help developers like you. If you have any suggestions for improvement, message me on LinkedIn.
If you enjoy this article, please support me by following me on GitHub and Twitter.
Thank you, and have a great day. 🫶
References
[1] Lifecycle definition & meaning from Merriam-Webster: https://www.merriam-webster.com/dictionary/life%20cycle
[2] The activity lifecycle: Android Developer Official Documentation: https://developer.android.com/guide/components/activities/activity-lifecycle
[3] App Anatomy and Lifecycle: App Development With Swift page 456
[4] Transition to the Suspended State: Handling Common State Transitions: https://developer.apple.com/documentation/watchkit/life_cycles/handling_common_state_transitions#3740560
[5] UIApplication Delegate: Apple's Official Documentation: https://developer.apple.com/documentation/uikit/uiapplicationdelegate
[6] application(_:didFinishLaunchingWithOptions:): https://developer.apple.com/documentation/uikit/uiapplicationdelegate/1622921-application
[7] App Anatomy and Lifecycle: App Development With Swift page 458
[8] applicationWillResignActive(_:): https://developer.apple.com/documentation/uikit/uiapplicationdelegate/1622950-applicationwillresignactive
[9]applicationDidBecomeActive(_:) https://developer.apple.com/documentation/uikit/uiapplicationdelegate/1622956-applicationdidbecomeactive
[10] App Anatomy and Lifecycle: App Development With Swift page 460
[11] applicationDidEnterBackground(_:) https://developer.apple.com/documentation/uikit/uiapplicationdelegate/1622997-applicationdidenterbackground
[12] App Anatomy and Lifecycle: App Development With Swift page 459
[13] applicationWillEnterForeground(_:) https://developer.apple.com/documentation/uikit/uiapplicationdelegate/1623076-applicationwillenterforeground
[14] applicationWillTerminate(_:) https://developer.apple.com/documentation/uikit/uiapplicationdelegate/1623111-applicationwillterminate