Swift 6: Typed Throws
Typed Throws
Swift 6 was released in September 2024 [1]. It introduced new testing frameworks, such as Swift Testing, and improved other fields, such as concurrency.
Also, with this new update, Swift has introduced Typed Throws.
Before I explain how you can use it, let's go over what a throw is in the first place.
Using Throws
Swift and many other languages provide ways to return errors without making them implicit in the return statement.
Let's take a look at the following example.
Someone asks you to bring a book from the library.
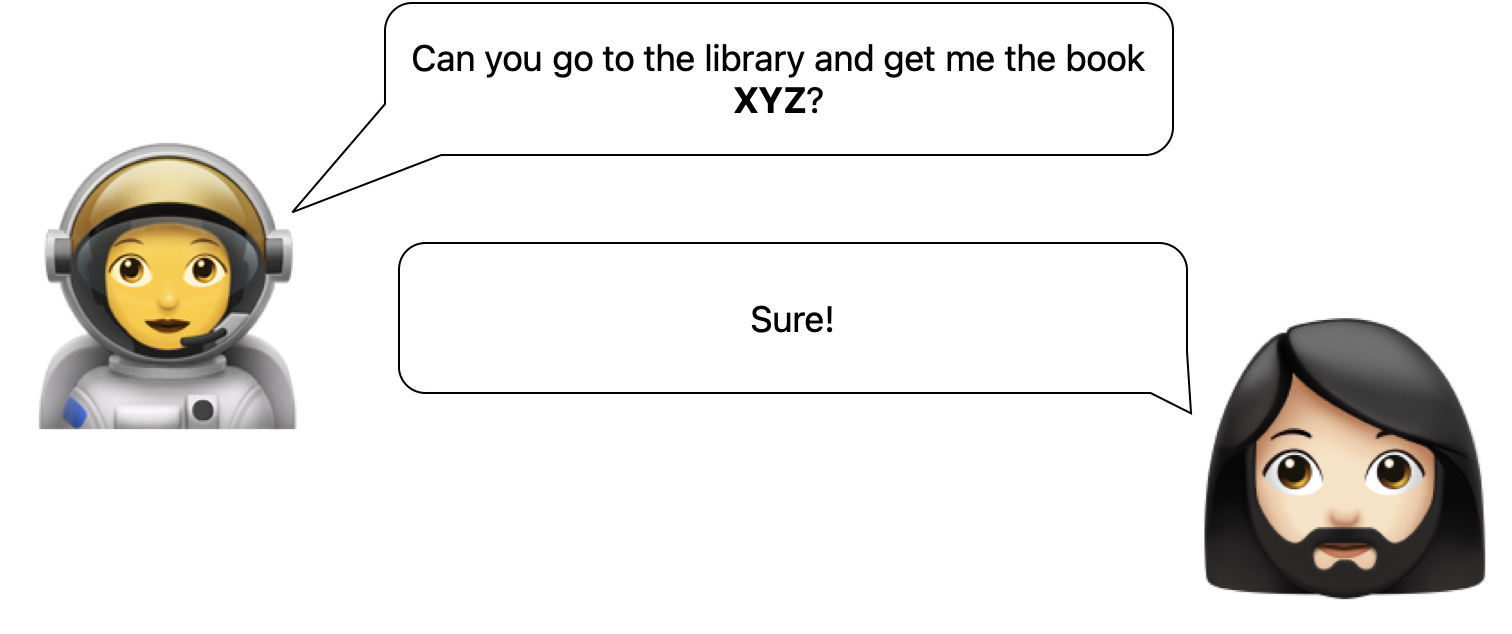
You notice that the book is unavailable when you go to the library.
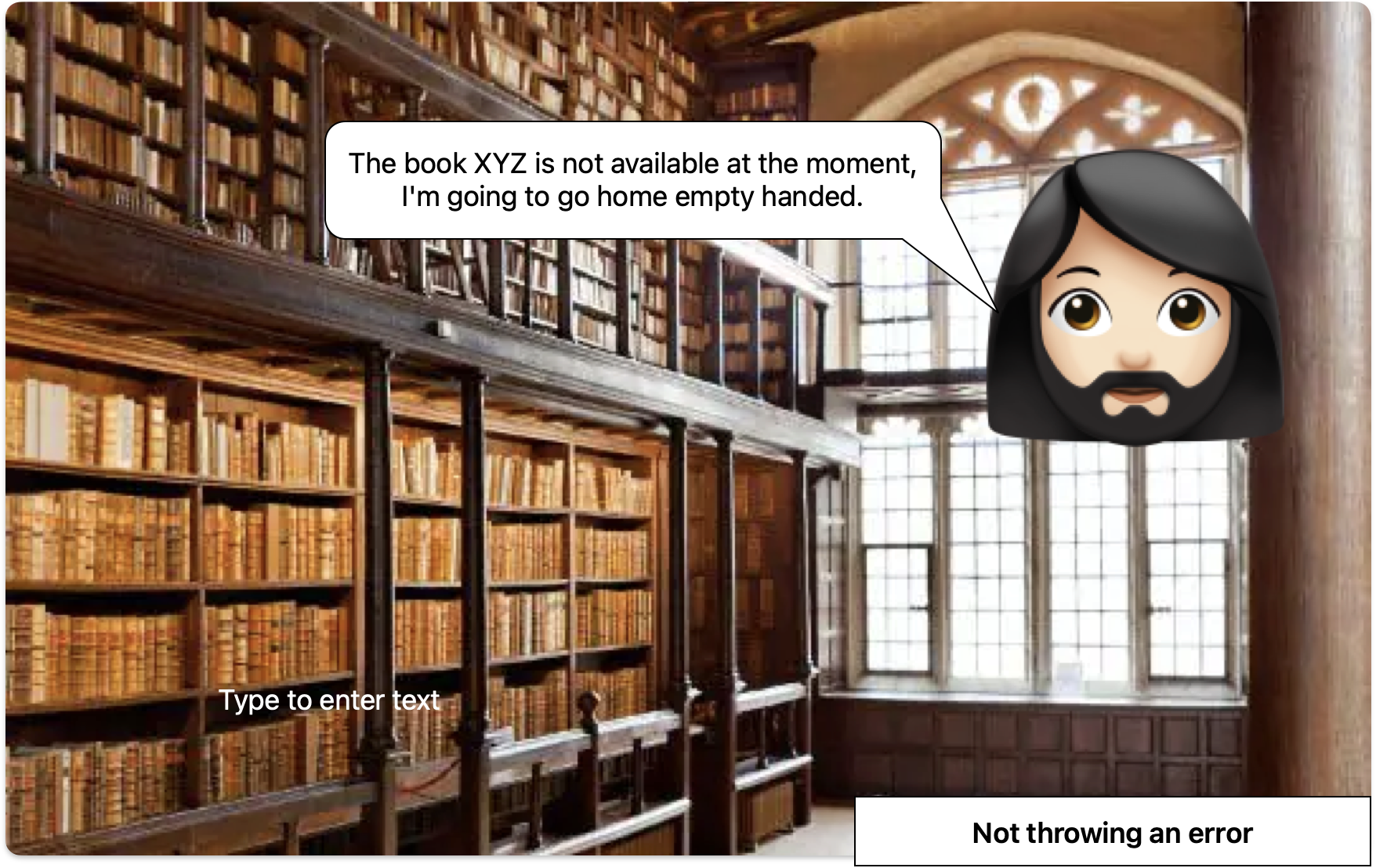
Now you come back and return with nothing.
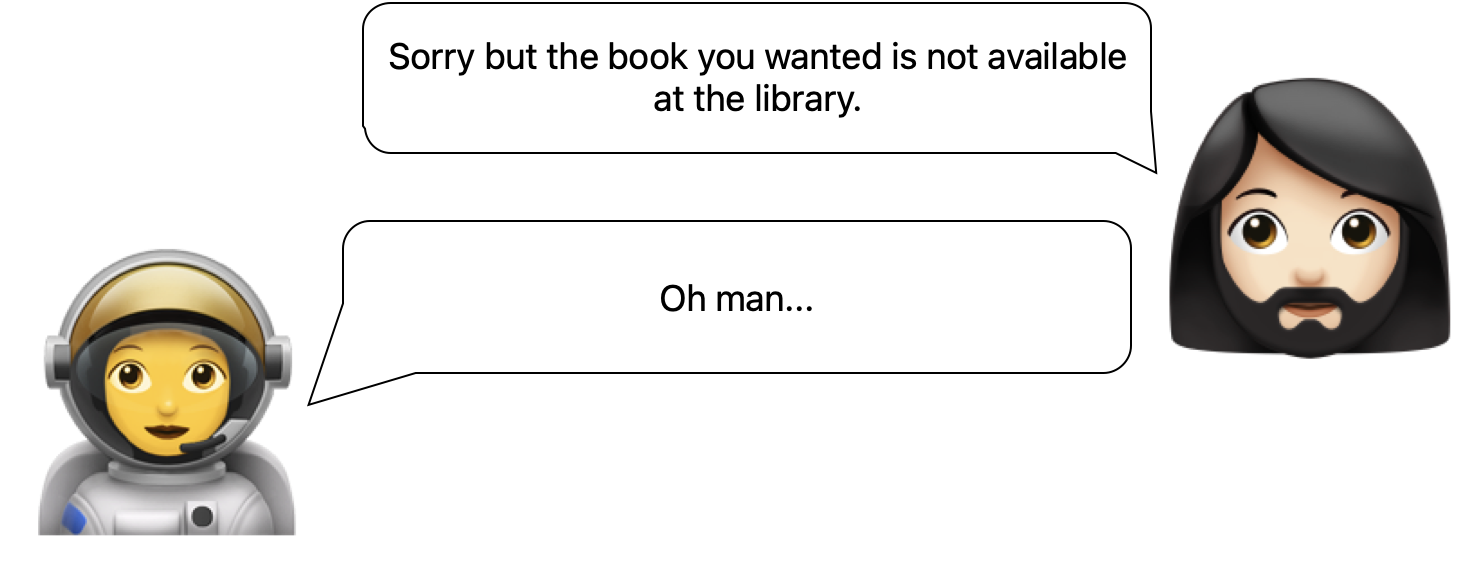
This is the typical flow of a function: do something and return something in the end.
However, you could send a message as soon as you found out the book didn't exist in the library, right?
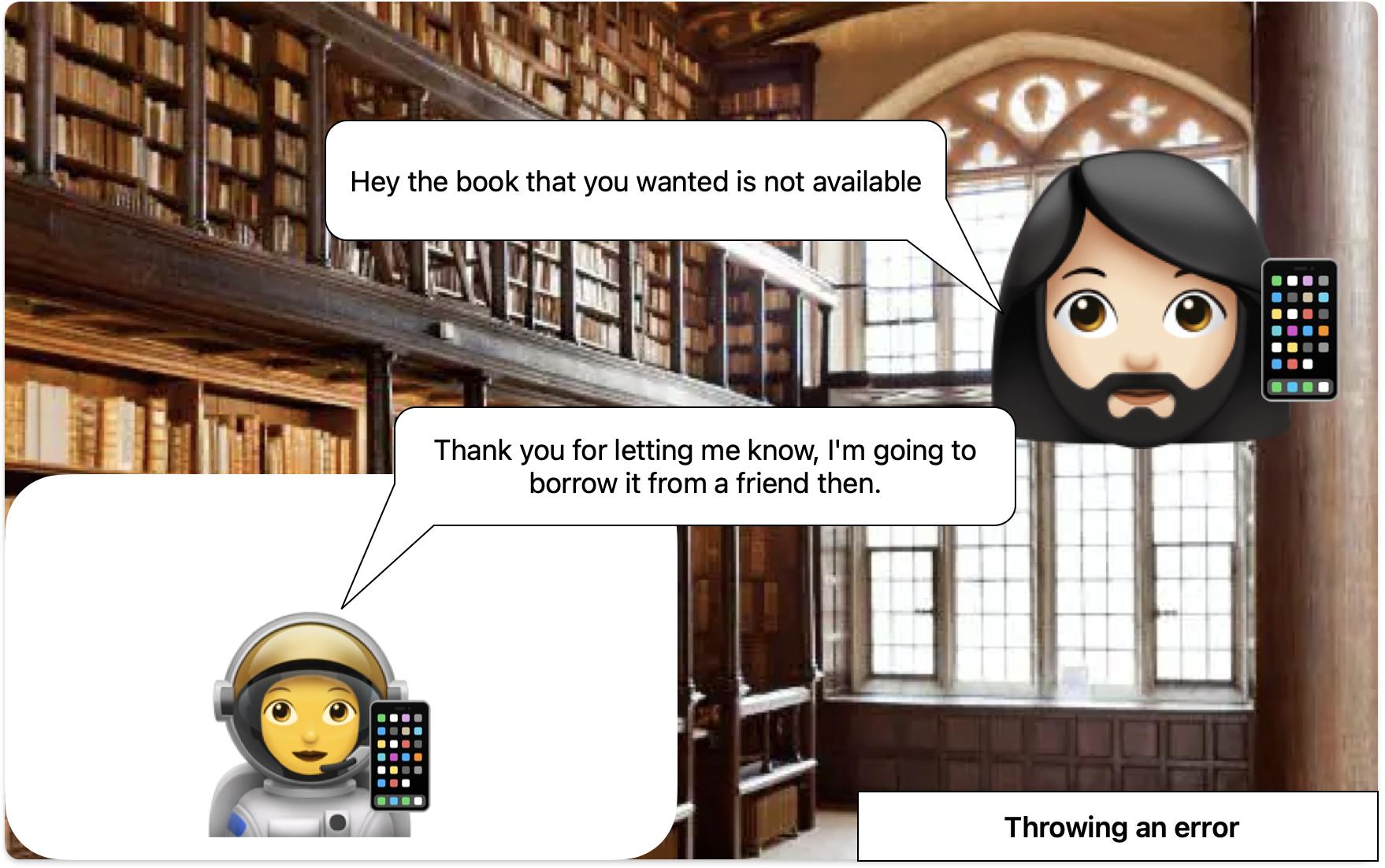
The notifying earlier than expected is what we call throwing an error.
Here's what Swift's official documentation says:
"Use throw to throw an error and throws to mark a function that can throw an error. If you throw an error in a function, the function returns immediately and the code that called the function handles the error [2].”
In Swift, if you want a function to return an error, you can mark the function with the throws keyword. Here's an example:
func readFileContent(at path: String) throws -> String {
...
}
At this stage, it's important to note that you can only throw something inherited from the Error protocol [2].
Handling the Error
Now that we have dealt with the concept of throwing, what's left?
When you throw an error, you need a place in the code to catch it. This is not mandatory, but if you don't catch an error, the compiler will catch it instead and do nothing with it.
You can handle the error in many different ways that I'm not going to go over in this article, but one example is to use do catch
block when you're calling a function that throws an error.
Where Typed Throws Come In
Up until Swift 6, you only needed to add the throws
. With this new update, you can specify the error you're throwing in a function.
So, instead of just adding throws
, you can specify the class of the error you will send.
func readFileContent(at path: String) throws(FileNotFoundError) -> String {
...
}
Why is this helpful?
If you can specify which type of error you can throw, it will be easier to deal with that error.
Before you would do something like this:
do {
let fileContent = try readFileContent(at: "path")
} catch FileNotFoundError {
print("File was not found.")
} catch error {
print("Something happened.")
}
This means that you can simplify the code of how you're going to handle the errors:
do {
let fileContent = try readFileContent(at: "path")
} catch FileNotFoundError {
print("File was not found.")
}
You can also go one step further and specify which error you will send.
enum FileNotFoundError: Error {
case whileReadingFile
case whileSearchingForFile
}
func readFileContent(at path: String) throws(FileNotFoundError.whileReadingfile) -> String {
...
}
If, for any reason, you throw a FileNotFoundError but not the one you want, the compiler will throw an error.
Taking the example of the book and the library that I gave before, if you didn't find the book, you could send a message with an SOS
.
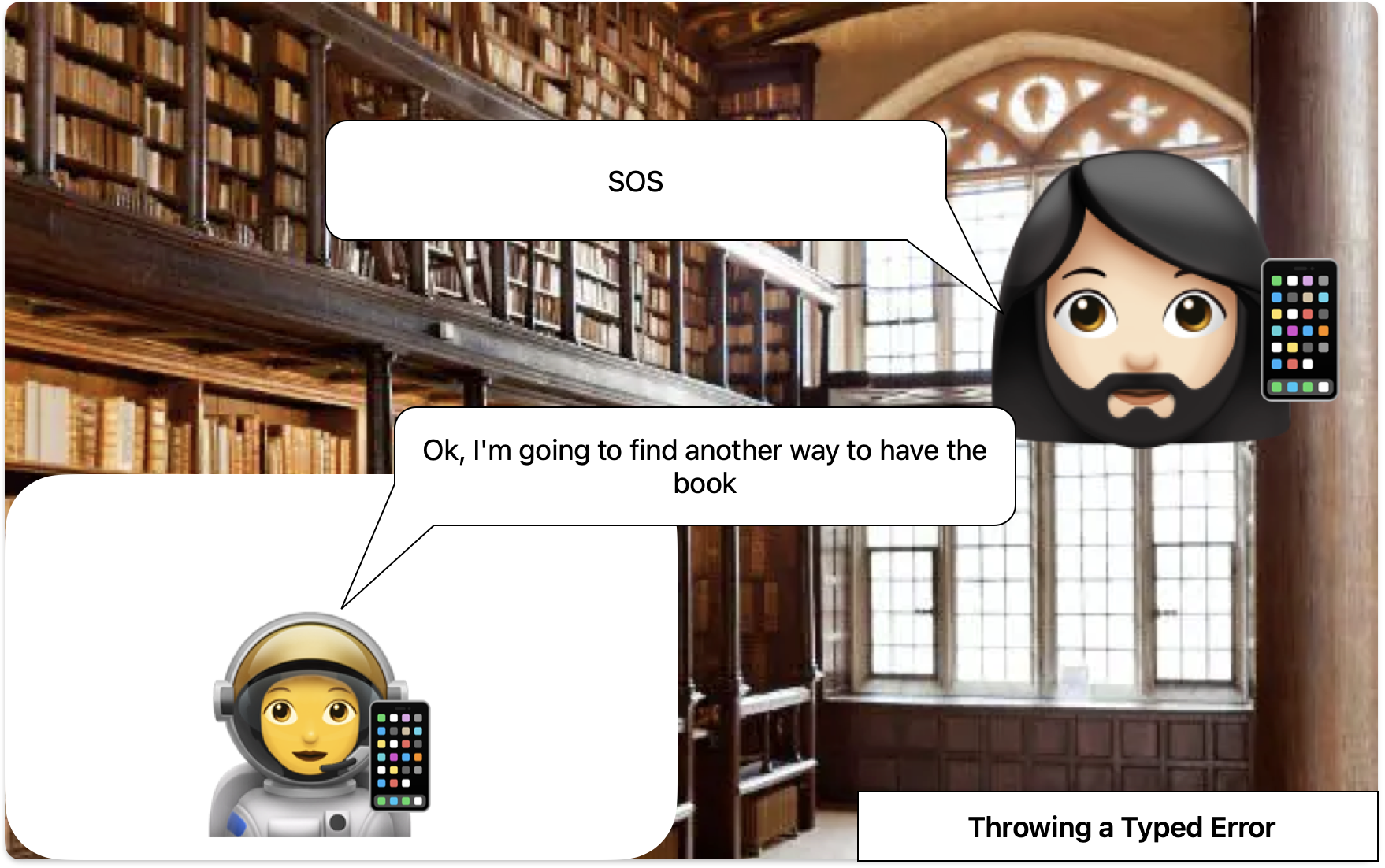
This would be enough for the person waiting for you to know that the book was unavailable.
Conclusion
Swift 6 has many new and exciting features, and now, you can be even more specific about the errors you are throwing. By specifying the type of error, you are sure that if anything goes wrong, the compiler can help detect the problem.
References
[1] Swift 6 release: https://github.com/swiftlang/swift/releases/tag/swift-6.0-RELEASE
[2] Error Handling: The Swift Programming Language (Swift 5.7), Apple Inc.
[3] Swift 6 Release Notes: https://www.swift.org/blog/announcing-swift-6/
Answering Your Questions
Today's topic comes from Alex. It's about keeping yourself up to date without getting overwhelmed.
Here's the question from Alex:
I'm an iOS Developer and it can feel overwhelming trying to keep up with all the new things popping up. There’s always some new tool or framework, and it’s easy to feel like I’m falling behind.
How can I stay up to date with the latest trends and technologies in a manageable way, without getting completely overwhelmed?
Software engineering is a very competitive field with many new technologies. I agree that it is very easy to be outdated. I also can't find the time to study 100% of the time.
For me, the answer is balance. I try to learn something new about Swift/iOS every week/ month. Keeping track of my goals and making them easy to accomplish makes my life easier.
Also, if you want to study new topics but can't afford a course, how about building an app with the latest technologies you want to learn?
That way, you build something you are excited about and have a reason to learn new things.
Thank you for your question, Alex. 🙂