Swift 101: Protocols
Introduction
When I started college, I was introduced to a completely new concept: interfaces. We were using Java at the time, and I quickly realized how useful they could be.
In programming projects, we used interfaces to create different domain objects that shared some similarities but also had their unique differences.
Everyone starts as a beginner, so I thought it’d be helpful to review what a protocol is in Swift. Whether you’re just starting out or have been an iOS developer for years, consider this a quick refresher.
Protocol vs Interface
Building on the previous example—in Java, we have something called an interface.
I won’t dive into that here since I want to focus on Swift, but keep this in mind: an interface in Java and a protocol in Swift are essentially the same thing.
Both are what we typically refer to as abstractions.
When you create an abstraction, you’re simplifying something by breaking it down into its common components.
Take a look at the definition of abstraction from Wikipedia:
In software engineering and computer science, abstraction is the process of generalizing concrete details (...) to focus attention on details of greater importance [1].
Also, we can say that a protocol (in Swift) is a contract you can establish between two objects. Here's Swift's official definition:
A protocol defines a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality [2].
Protocols are helpful in establishing a connection between objects and, especially, establishing the flow of data between them.
Example
So, after we dive into the boring definition, I want to talk about why protocols are so important and why they're universal between programming languages, especially object-oriented languages.
Let's look at the following example:
Imagine someone asking you to count the number of hands in a room. It's a weird request, but let’s roll with it.
Step 1: What counts as a hand?
First, we say a hand must have extremities (fingers).
So... does a dog have four hands? Nope, that doesn’t feel right.
Step 2: Be more specific.
Okay, let’s say it must be attached to a human.
But wait—if we include feet, humans have four extremities, too (two hands and two feet).
Step 3: Narrow it down further.
Let’s add another rule: a hand is something you can hold things with.
Sure, some people can use their feet to grab stuff, but for now, we’re focusing on the usual idea of hands.
We could keep refining this forever, but you get the gist. After reading this article, feel free to explore more differences between hands and feet.
We’ve now created a mental “protocol” for defining what counts as a hand—it’s like a contract we’ll use for our counting.
Using this protocol, take a look at the following illustration. How many hands would you count?
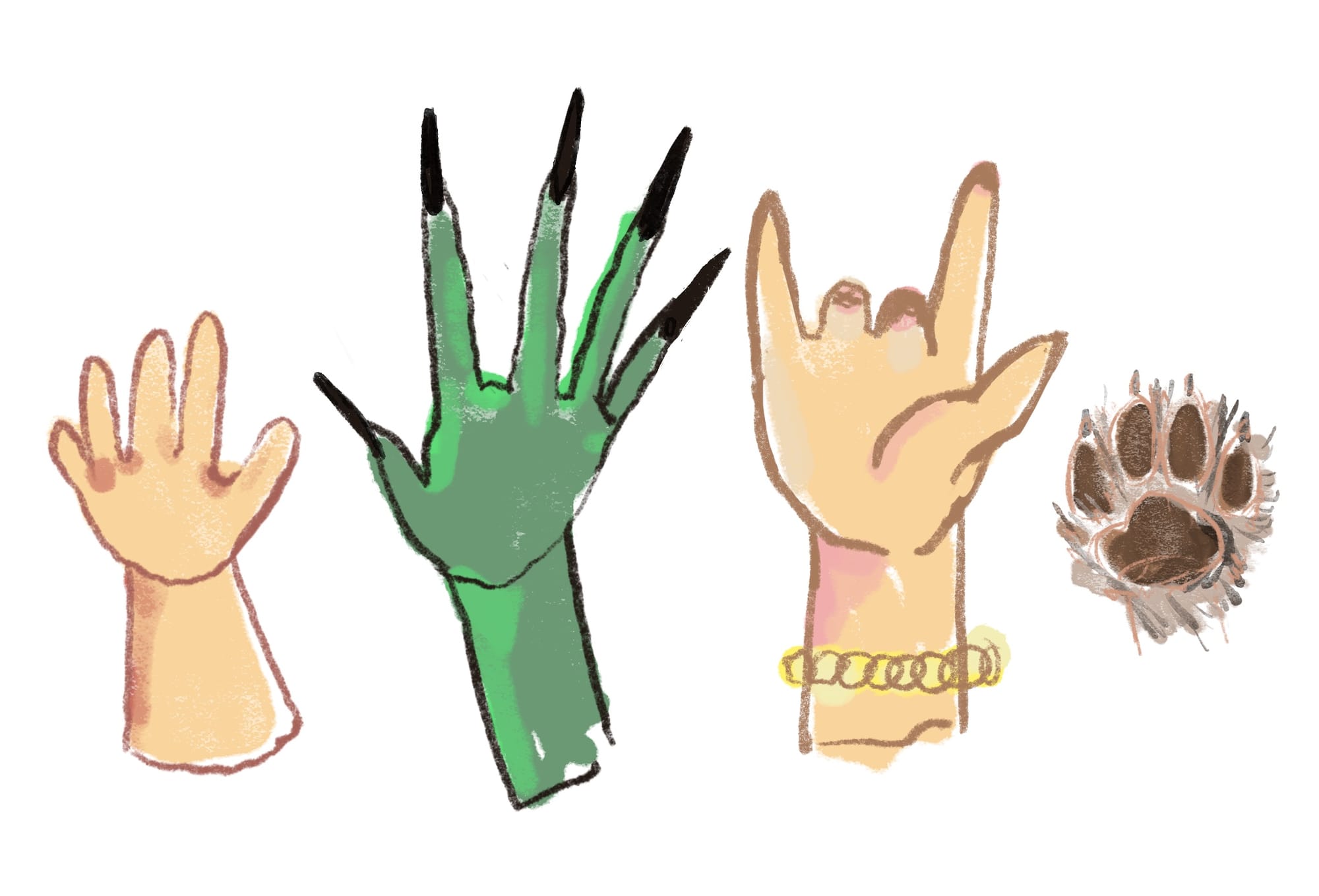
Conclusion
Now that you know more about protocols, you can see how and where to use them. In Swift, protocols can be adopted by classes, structures, and enums, making them incredibly versatile. By establishing a common component across different elements, protocols simplify passing data and improve code organization.
Answering Your Questions
Today's question comes from Hanna:
Hey Vera, quick question for you! Working as an iOS Developer can be pretty tough, especially when you're on the hunt for a new job. How do you make yourself stand out when applying for positions? Any tips or tricks you swear by?
To land a great iOS developer job, you must stand out with a strong portfolio, polished CV, and excellent presentation. Your GitHub is crucial to showcase your abilities to other developers and recruiters.
Review your LinkedIn for errors or inconsistencies and view it as if you were a stranger. Ask colleagues for feedback on your CV and profile to catch things you might miss.
Publishing apps on the App Store is a big plus and often a requirement for senior roles.
Most importantly, keep refining and improving your materials—it makes all the difference!
Thank you for your question, Hanna!
References
[1] Abstraction: Wikipedia: https://en.wikipedia.org/wiki/Abstraction_(computer_science)#cite_note-:1-1
[2] Protocols: Swift: https://docs.swift.org/swift-book/documentation/the-swift-programming-language/protocols/